Tuesday, November 25, 2008
AskWise v1.0.0
I finally finished rewriting AskWise and it think it's time to take it out of beta. It is a menu driver console application that using databases with sets of known numerical data, predicts missing values in other sets of numerical data. For example, one of the included databases has some sets of height, weight, age and sex (male=0 female=10). Given this database, AskWise can predict someones sex given his height, weight and age. In fact you can give it whatever values (of these four) you know for a person and it will try to predict the rest of them. This database is not useful but you can create your own databases and use them with AskWise.
That's the fourth time I program AskWise's algorithm. The first one was during my final year in school when me and a friend of mine were challenging each other to find functions with specific strange graphs. I though I should try to make a function that can easily be adapted to connect any number of points, so I made one. Later I realized that I could use this function as a quick and dirty way to predict missing values in a set so I wrote a program in PLua that used it for this purpose. I later rewrote it in Visual Basic improving the algorithm a little. Then I rewrote it in Lua, further improving the algorithm and now I wrote it again in Lua, using almost the same algorithm but with a better, menu driven UI.
The new version is released under the terms of the GNU/GPLv3, works on both Windows and Linux and can be found here.
I also wrote some documentation for it, where I try to explain both how to use AskWise and how it makes it's predictions. It was quite hard to do this in English and I believe that my syntax might be quite complex in some parts. If you believe that something is lacking clarity (I'm sure there are such parts) then, please, point it out to me.
PS: This is out of beta but there might still be bugs. If you find one, AskWise will create a small bug report on it's directory, I would be glad if you send it back to me. :-)
Thursday, November 20, 2008
Long absence (or: my news)
I also work on another program that produces an exam period schedule based on what subjects each student will take an exam on, so that students have enough days between the subjects to study. It works very well but, although it scales linearly, I find it quite slow for big input data, roughly estimating that it will need 2 days to optimize the exam schedule for a school of 3000 students. So I am still working on improving it's speed. I will post this program here when I finish it.
I am also rewriting AskWise from the scratch. It will be a menu driven console program and I hope that I won't consider it a beta anymore when it's finished.
Finally I was going to rewrite for the new Intrepid Ibex my old Vaio & Hardy Heron how-to but I don't have to, since Shawe wrote an excellent how to. It is in Spanish but you can easily understand it even without translating it.
So I guess my next post will be in less than a month. :-)
Have fun.
Sunday, September 14, 2008
Tiny Lua Distribution 5.1.4
Thursday, July 31, 2008
Big table implementation in Brainfuck
Also, all known implementations had a limit of 256 cells (Or equal to the size of a cell on the Brainfuck array. For the rest of the post I will assume that the cell size is one byte).
During my road-trip to Spain, based on my old implementation, I found a new one that can use multiple indexes to implement tables of ANY size on the Brainfuck array. Additionally the table cell size can be any multiple of the Brainfuck array's cell size.
It uses a format similar to my old implementation: a head moving through the table while decreasing the index it carries, passing table data over it, until it finds the correct position,the it does it's job (read/write) and then it returns in the same way but using a copy of the index.
For a table with n cells of size k each the form of the header is:
- k empty cells: When the head need's to move to the right, it will have to move the first k cells on it's right in these empty cells before moving itself k positions to the right (thus moving itself to the next indexed position)
- n index cells: Before starting a read or write operation you will have to store the index here. The leftmost index is the most important. For example if the maximum possible value for an index is 9 (instead of 255) and you use two indexes, to access the 15th cell you would have to use 1 for the left index and 4 for the right index (not 5 because the numbering starts from 0).
- k data cells: To write to the table you will have to put the data in these cells. If you read from the table, after the end of the read operation the read data will be here. These cells MUST be cleared before starting a read operation.
- n empty (index) cells: While the index cells are reduced, these cells are increased so that, when the seek operation finishes, they will hold a copy of the original indexes which will be used for returning the head to the beginning of the table.
After the header the table data is assumed to be laying. n*k cells in total. For example for a size 400 table with 2 bytes per cell they would have this form:
Data 1 of index 0,0
Data 2 of index 0,0
...
Data 1 of index 0,255
Data 2 of index 0,255
Data 1 of index 1,0
Data 2 of index 2,0
Data 1 of index 1,1
Data 2 of index 2,1
...
...
Data 1 of index 1,143
Data 2 of index 1,143
So you will need 2*k+2*ceil(log256(n))+n*k cells on the Brainfuck array to store a table with n, k-sized cells. (ceil(log256(n)) is the number of the indexes)
Therefore the code needed to write or read from the table depends on n and k. I actually wrote some metacode that provided with n and k produces the corresponding code. I then turned the metacode into a Lua script. It's not well written but I believe that it works. I tried the following cases:
- Writing two values to a table with n<256 and k=1 and then reading them back.
- Writing two values to a table with 256<n<256^2 and k=2 and then reading them back.
If you want to try the script yourself you download it from here.
Here is some example code:
n<256 k=1
Write:
>[->>+>[-<<<<+>>>>]<[->+<]<[->+<]
<[->+<]>]>>>[-]<<[->>+<<]>[-[-<+>
]<<<<[->>>>+<<<<]>>>]<<<
Read:
>[->>+>[-<<<<+>>>>]<[->+<]<<[->+<
]>]>>>[-<<+<<+>>>>]<<<<[->>>>+<<<
<]>>>[-<[-<+>]>[-<+>]<<<<[->>>>+<
<<<]>>>]<<<
256<n<256^2 k=1
Write:
>>[->>>+>[-<<<<<<+>>>>>>]<[->+<]<
[->+<]<[->+<]<[->+<]<[->+<]>>]<[-
>>>+>>[-<<<<<<+>>>>>>]<[->+<]<[->
+<]<[->+<]<[->+<]<[->+<]>>-[->>>>
[-<<<<<<+>>>>>>]<[->+<]<[->+<]<[-
>+<]<[->+<]<[->+<]>>]<]>>>>>[-]<<
<[->>>+<<<]>>[-<[-<+>]>[-<+>]<<<<
<<[->>>>>>+<<<<<<]>>>>>]<[-[-<+>]
>[-<+>]<<<<<<[->>>>>>+<<<<<<]>>>>
>-[-<[-<+>]>[-<+>]<<<<<<[->>>>>>+
<<<<<<]>>>>>]<]<<<<
Read:
>>[->>>+>[-<<<<<<+>>>>>>]<[->+<]<
[->+<]<<[->+<]<[->+<]>>]<[->>>+>>
[-<<<<<<+>>>>>>]<[->+<]<[->+<]<<[
->+<]<[->+<]>>-[->>>>[-<<<<<<+>>>
>>>]<[->+<]<[->+<]<<[->+<]<[->+<]
>>]<]>>>>>[-<<<+<<<+>>>>>>]<<<<<<
[->>>>>>+<<<<<<]>>>>>[-<<[-<+>]>[
-<+>]>[-<+>]<<<<<<[->>>>>>+<<<<<<
]>>>>>]<[-<[-<+>]>[-<+>]>[-<+>]<<
<<<<[->>>>>>+<<<<<<]>>>>>-[-<<[-<
+>]>[-<+>]>[-<+>]<<<<<<[->>>>>>+<
<<<<<]>>>>>]<]<<<<
Saturday, July 5, 2008
Windows XP and Vista running on Ubuntu
Still not switched to Linux? ;-) You won't miss Windows this way...
PS: The FPS aren't that slow as they seem to be on the Video. Also, some horizontal red stripes that appear while rotating the cube are a glich in the capture and don't really exist.
Monday, June 23, 2008
Windows 2.03 on a Virtual Machine
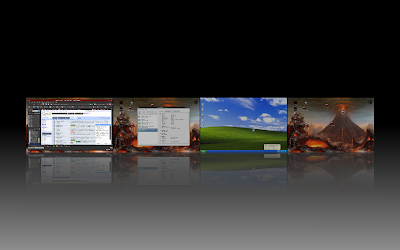
Apart from running a "useful" OS on the virtual machine (it's useful because I needed to use a 3G GPRS USB modem which doesn't work on Linux) you can also run old or rare OSes. I tried Warp but I couldn't install it just like Windows 1.0. So I tried Windows 2.03 which did install and run properly. I also made a video with a quite lengthy tour showing most of their programs. (it's five minutes long!)
Here it is:
Saturday, June 14, 2008
Hanoi Towers Solver
Thursday, June 5, 2008
Ubuntu Hardy Heron on a Sony Vaio VGN-FZ31E
Yeap I bought it. I though that since it had an NVidia card and it was Centrino based etc it wouldn't have any incompatibilities with Linux. (aaaargh if I only knew...) Anyway.
The laptop came with Vista. I was going to keep them for a week or so just to make sure I don't miss any of the Sony specific programs that was preinstalled. Well I couldn't bare them for more than 24 hours. I made some factory restore CDs and repartitioned the whole drive which now only has Ubuntu installed in it.
What worked out of the box:
The SD/MS reader (104c:803b). It works out of the box with SD cards but the MS PRO slot doesn't work for me and I have found no way to make it work.
Graphics Card (10de:0426): The NVidia GeForce 8400 GT worked perfectly with the closed source drivers that Ubuntu installed. The temperature sensor works OK too.
Nevertheless I recreated the xorg.conf (for no specific reason). Here is the new one.
DVD-Drive (PIONEER DVD-RW DVRKD08): It works OK. I recorded a data DVD with Brasero (for some reason it couldn't verify the burned disk afterwards but it worked perfectly). I also installed Diablo 2 through Wine. The CD protection of Diablo 2 works OK and recognizes the original CD.
CPU (Intel(R) Core(TM)2 Duo CPU T5450 @ 1.66GHz): Both cores are recognized and the temperature sensors work OK. The same goes for frequency scaling.
Wireless and LAN (8086:4229 and 11ab:4351): They both work perfectly right out of the box.
What didn't work out of the box but works with some tricks. Before trying the tricks enable the extra repositories (universe, multiverse etc) from System->Administration->Software sources).
Camera (05ca:183b): HAL loads a wrong module for the camera that in addition to not working, makes the laptop crash when you try to suspend it. So you must remove the misbehaving module from the kernel and prohibit reloading it.
- Type the following in a console (you can find the console in Applications->Accessories->Terminal):
sudo gedit /etc/pm/config.d/unload_modules
Give your password and then copy and paste the following line at the end of the file:
SUSPEND_MODULES=uvcvideo
Save the file and close the text editor. - To download the r5u870 drive you will need svn, so in a console type:
sudo apt-get install subversion
(answer yes to any questions you may encounter) - To download the source of the driver, type in the console:
svn co http://svn.mediati.org/svn/r5u870/trunk r5u870 - Now that all files are downloaded go into their directory by typing:
cd r5u870 - Install the build-essential package before trying to compile the drivers by typing:
sudo apt-get install build-essential - Compile the driver by typing:
make - and then install the driver by typing:
sudo make install - Now instead of restarting you may just load the module into the kernel by typing:
sudo modprobe r5u870
When you reach this point the camera will only work with some applications like Skype.
If you open Cheese (a very nice application for capturing video and still pictures from your camera, install it by typing sudo apt-get install cheese) you will notice that it doesn't recognize your camera. That's because the system doesn't recognize it as a Video4Linux camera. - To fix this you will have to download this file and copy it to /usr/share/hal/fdi/information/20thirdparty . If you don't know how to do this just type the following two lines in the console:
cd /usr/share/hal/fdi/information/20thirdparty
sudo wget https://bittit.info/publicDro/10-r5u870-webcam.fdi - Now either restart you computer or execute this in the console:
sudo /etc/init.d/hal restart
Now you should have a perfectly working camera! Oh, you may now delete the sources by typing:
sudo rm -r ~/r5u870
Sleep and Hibernate: They don't work initially. The computers go to sleep but when awaken it stays in a black screen. Fixing the camera will fix this problem too. :-)
Sound Card (8086:284b): Well, playback worked out of the box but when opening the sound options there were no recording controls and the sound was coming out only through the builtin speakers, plugging in headphones changed nothing. To fix all these problems follow these simple steps:
- Optional step. I suggest that you don't use Pulseaudio because I found it a bit buggy. To switch back to ALSA go to System->Preferences->Sound and change everything to ALSA.
- Type the following in a console:
echo "options snd-hda-intel model=vaio" sudo tee -a /etc/modprobe.d/snd-hda-intel - Restart you computer.
- Open the sound controls by clicking the sound icon next to the clock.
- Select File->Change Device->HDA Intel (Alsa mixer)
- Then goto Edit->Preferences and select everything.
- Play around with the controls. Don't forget to look at all three tabs (in the third tab you will find the record selection switches: Internal Mic, PCM, Mic Jack)
Bluetooth (044e:3010): It is properly recognized by the system but it will not work. Here is a way to fix it:
- In a console type:
sudo gedit /etc/rc.local - Now append the following line before the exit command (if there is one) near the end of the file:
hciconfig hci0 reset - Now save the file and close gedit.
This way the bluetooth will work correctly but if you switch it off and again on (using the hardware switch in the front side of the laptop) it will stop working so you also have to do the following:
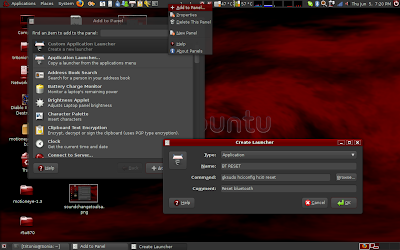
- Right click on an empty space on your panel and select "Add to panel..."
- Then create a new custom launcher and in the command textfield copy and paste this:
gksudo hciconfig hci0 reset
VGA-out:
Open a console and type:
cp /etc/X11/xorg.conf ~/xorg.conf.back
This will back up your xorg.conf file just in case you mess it up.
Now you will have to install the NVIDIA settings manager. To do this just type:
sudo apt-get install nvidia-settings
And then run it by typing:
sudo nvidia-settings
The NVIDIA settings manager will open. (you might need to resize this window because for some strange reason it initializes too small)
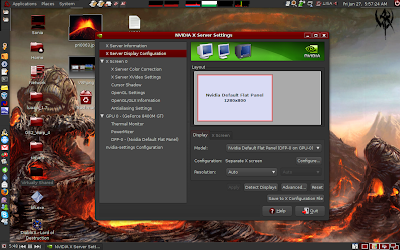
Now select X Server Display Configuration and then click on Detect Displays to detect the connected display. Now you will be able to configure the two screens using the options in the Display and the X Screen tabs.
From ere you may also set the resolution to for your laptop's monitor to the correct one (1280x800). If you select a resolution from the Resolution drop-down-list (it's visible in the above picture) the drop-down-list on its right will be enabled and you can then select the desired refresh rate.
Afterwords don't forget to click on Save to X Configuration File.
If you mess up then type this in a console to restore your backup:
sudo cp ~/xorg.conf.back /etc/X11/xorg.conf
To delete your backup type:
rm ~/xorg.conf.back
Tip: To get rid of the annoying NVIDIA splash screen type this a a terminal:
sudo gedit /etc/X11/xorg.conf
Then find all the Device sections and add this line inside them:
Option "NoLogo" "True"
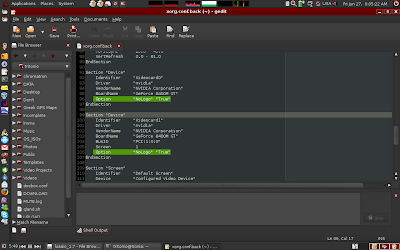
Then save and exit.
What doesn't work and still I have found no solution:
The Fn buttons don't work. In fact the sound controls work, the next track, previous track, play, stop buttons work too. The S1 (customizable button), the AV MODE and the brightness control buttons doesn't work. I am still looking for a solution. The MS PRO slot doesn't work and I still haven't found any solution.
What I haven't fully tried yet:
S-Video out, HDMI output. I'll try them some time... (Shawe in the comments says that HDMI works OK but without any sound.)
References:
http://ubuntuforums.org/showthread.php?t=706530
https://bugs.launchpad.net/ubuntu/+bug/147757
http://wiki.mediati.org/Installation
https://launchpad.net/ubuntu/+source/alsa-driver/+bug/33719
PS: If I fix more problems I will update this post so you might want to check back later. If you find any problems or omissions please leave a comment.
PS2: It seems like someone did a partial translation of this article into Catalan. Here is a link to it. :-)
PS3: Thank you for your comments and the info you provided!
Monday, June 2, 2008
ZVista
It's sooooo sloooooooowwwww..... I have friends with Vista on their laptops, some of them just use them for office and internet, not a single game or other application. Well it takes around 10 minutes to do a restart! Before some days I was waiting 7 minutes for the shutdown before forcing a power off myself! (no it wasn't even installing updates)
Apart from the speed issues there are million other problems. It's amazing how they managed to mess up every single aspect of the OS. Even the console (the command prompt) doesn't support drag and drop!!! WHY??? Are they crazy? I mean, did they actually pay someone to do this??? In addition to this the changed the layout of the start menu to a more flat one. They also made changes to Windows Explorer. I was looking into a folder containing C source files with the details view mode, well, Vista insisted to show me artist name, album, etc for every source file! For some unknown reason it though this was a music folder! (Perhaps it wouldn't do so if there was a Visual C++ project file in the directory >:-P ) All of the above make Vista the worst OS for a programmer or even someone that wants to do more than surfing and writing in Microsoft Office!
Another really stupid aspect is that dialog that pops up every time you try to alter system files. I really like the extra security that it offers but a virus managed to infect a system without even triggering this dialog! (I accidentally run the virus on a friend's computer) So no extra security actually, just extra confirmations to get on your nerves.
Vista is really a stupid OS for stupid (trendy) people that want to see glossy windows, but even their 3D effects are inferior to what Compiz offers to Linux users (and of course Aero needs even better hardware).
The funny thing is that I am planning to buy a new laptop but they all ship with Vista preinstalled! Of course the first thing to do will be to get rid of them but I don't even like the idea that I will actually pay for this crap!
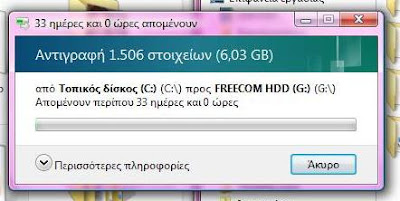
The dialog reads:
33 days and 0 hours remaining
Copying 1.506 items (6.03GB)
from Local Disk (C:) (C:\) to FREECOM HDD (G:) (G:\)
About 33 days and 0 hours remaining
Well, this was not Vista's fault (drive G: was problematic) but it describes, a bit dramatically, what you will go through if you use this NOS (Non Operating System)
Want some more?
Get a life...
PS: ZVista is pronounced like the word "delete them" or "clear them" in Greek.
Friday, May 23, 2008
Game of Life
I went to some stores but I couldn't find any IDE HD with more than 80GB capacity, so I'll wait one more day for their supplies to arrive. One of the salesmen told me that they "might" get some IDEs for laptops this Friday. >:-(
Anyway. Today I made a nice little prog that calculates a possible state in Game of Life that will evolve into a specific state after one step. I'm too tired to explain more about the Game of Life so try wikipedia. :-)
Here's what I made with it:
# ## ## # # #
# #
#### #### ##### #### ########### ###########
## # # # # # # # # #
### # # ## #### # # # # #
##### ## ## ## ####### # #
# # # ## ###### # ### # #
# # # ## ## ## ## # #
## ### # ### ##### # # ## #
# # # # ## ## # ## # # # # #
# ## # # # # # ## # # # # #
### # # ##### ### ### # ## #
## ## # ## ### # ### # # ## #
### ### # # ## # # # # #
#### # # ### # ## # # # # #
# ### ######## ######
# # # ##### ####### ## #
To the left is the first state which evolves into what you see to the right. (TrT stands for Tritonio). I simply gave the right state to the program and it calculated the left one. The script uses an algorithm similar to the one I used while making my keyboard.
I am trying to make an "INSHAME" logo right now but, if at all possible, it will take much time due to it's size.
If you are really curious you can find the Lua source code of the program here but it's undocumented, written in the past three hours, and geek-only. ;-)
UPDATE:
Finally the Logo has finished!!!!!!! Here it is!!!
# # ## ## # # # # ## # # # # ##
# # # # # #
### ### ### ##### ## ## ## # # #
## # #### # #### # # # ### # # # ####
# # # # # ## # # ### # # ## # #
## # ## # ## # ### # # ## # # # #
## # # ### # ##### # # #### ##
## ## # ## # # # ## # # ###
# # # # #### # #### ### ##
# ### # # ## # ### # # # ### #### ## #
# # # # # # # ## # ##
# # # # # # ## # # ## # #
This evolves into INSHAME! :-D
I am still improving the algorithm.
Friday, May 16, 2008
Isn't it ironic?
People cry for the 80 that died from the fires in Greece.
People cry for the 2948 people that died in the WTC.
But people don't cry for her...
What's more tragic? Her and the other 9.7 millions kids per year (1 death per 3.25 seconds)? Or the 21 students? Don't tell me that tragedy cannot be measured. Because it can! Dying from starvation is not the same thing with dying in an accident... And 1 million kids are more than 100 kids.
People don't cry for the thousands of impending deaths because of the huge natural disaster that the fires caused. 2.600.000 acres burned leaving nothing but our future diseases... We will die of cancer, floods or even wars for water, but we mostly care about the 80 that died.
Every single death is a tragedy. I don't expect from someone who lost a friend or a relative not to mourn him because some kids in Africa die. But I expect from everyone else to stop being a hypocrite or illogical (whatever is the case).
If you feel like crying after seeing about the death of 21 kids on the TV and you don't cry about the hundreds that die at the same time, just because the TV didn't report it then you are really illogical. If you don't fell like crying but you still do then you are a hypocrite.
Next time don't cry when hearing about a tragedy on the TV, instead try to understand it logically. Because if you only confront it emotionally it will last very shortly and you will gain nothing from it.
Thursday, May 1, 2008
Ubuntu Hardy Heron 8.04
Story 1:
Installation:
I made a fresh install on my Acer Aspire 9420. Not a single problem! All of the devices were installed automatically and worked almost perfectly!
Customization:
The first thing I was going to do with Hardy was to install the programs that I used with Gutsy. I was really pleased to see some of them (Emesene, Qlandkarte*, Infon) included in the new repositories, as this meant more time to explore the new release. So I went on and added extra repositories: Ubuntu Satanic Edition (wonderful theme!), Medibuntu, Wine.
Satanic Edition Theme:
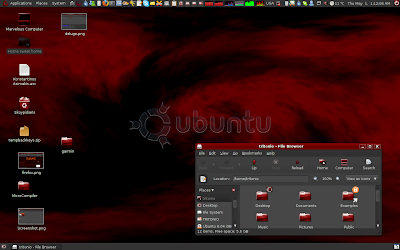
After that I started installing Add-Ons for Firefox. Hardy comes with Firefox 3 beta 5 installed. Although it feels much better than version 2, many of my favourite Add-Ons are not compatible with the new beta (yet...).
Firefox 3 beta 5:
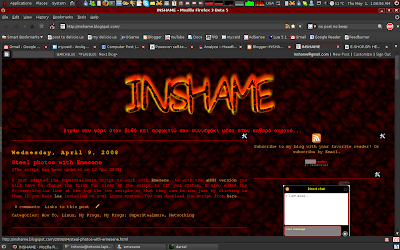
I also found a very nice bittorrent client called Deluge. Well, in fact I already knew about Deluge but it's the first time that I actually use it. ;-)
Deluge:
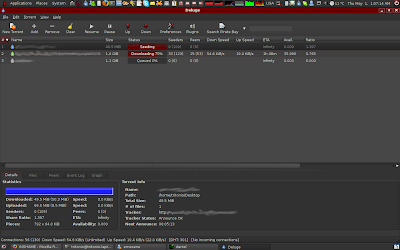
I was amused by how easily accessible are encryption and digital signing capabilities in Hardy. Well in fact you have to run seahorse to create your public-private keypair but after that you can simply use the right-click context menu and the Clipboard encryption panel add on.
How to add the Clipboard Encryption panel add on:
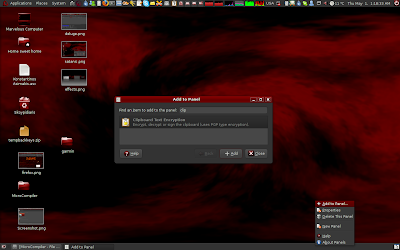
Seahorse is in the background, a digital signature in the foreground:
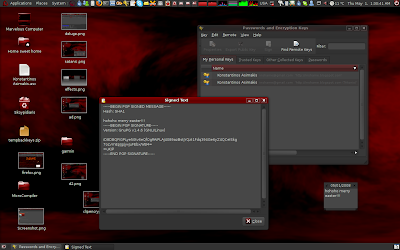
Finally I had to setup all the Compiz plugins once again because for some reason the backup of my old settings didn't seem to work with Hardy... :-( But the result is nevertheless rewarding. :-)
The four desktops as seen with the Expo plugin:
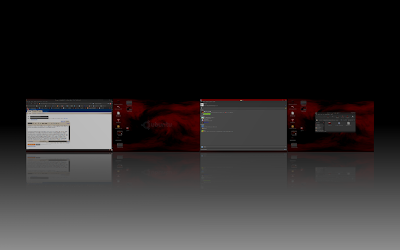
Story 2:
Installation:
I also decided to install Hardy on my father's PC. I had a serious problem that didn't allow me to run the live CD. After reading about it I managed to solve it by adding the all_generic_ide boot option. It seems that there a major bug in the Live CD but luckily it doesn't affect all PCs. After installing Ubuntu everything worked just fine.
Anyway. Well done to everybody that worked on Hardy! :-)
*(I am not sure if Qlandkarte wasn't available on Gutsy's repos)
Wednesday, April 9, 2008
Steal photos with Emesene
Monday, April 7, 2008
Emesene
Still there was some things in aMSN that kept annoying me.
- Offline messaging was too slow. After pressing enter I had to confirm that I want to send an offline message and then the whole program stopped responding for one-two seconds.
- The program sometimes crushed after long runtime periods. I have found in many occasions, after returning home, white aMSN windows which do not respond.
- The program feels a bit heavy. Well, maybe... But some things take too long and the program stops responding (login etc).
- There are many other minor bugs like false "file send failed" messages etc.
- Connection problems. Some times (quite often) I had to close and reopen a chat window in order to be able to send again a message to a contact. Else I would get "could not deliver message" errors (or something like this).
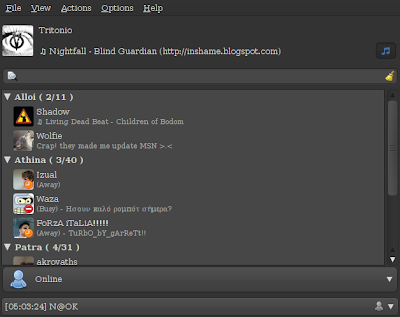
Now I am using Emesene (wikipedia). I found out about it from a guy in my University's forums. It misses some features compared to aMSN like camera and voice support) but I really don't care. After all I use Skype for these. On the other hand it's much lighter (written in Python), it responds really fast, no crazy crashes, and lots of neat plugins!
Initially all plugins are disabled. You have to enable them through Options->Plugins. Some notable plugins are:
- Youtube which shows thumbnails next to youtube links that your contacts send to you.
- Tinyurl integration.
- CurrentSong which changes you personal message to show the currently playing song and even supports Listen! (my favourite music player for Ubuntu)
Try it! It's by far the best MSN client I have tried!
PS: There is a Windows version too but I haven't tried it. A friend told me that it doesn't work for him.
Monday, March 17, 2008
Using aMSN to steal/archive contact photos
Tuesday, March 4, 2008
BMPGlyph
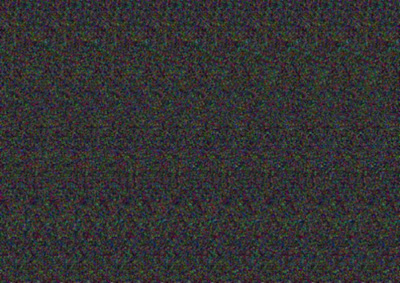
Do you know what is this? No, it's not some random picture. It's a stereogram, more specificaly it's a random dot, diverging, autostereogram. It's a 2D picture designed to trick the brain into believing that it is a 3D picture if viewed correctly.
There is at least one program for Linux that creates that kind of pictures but I decided to make my own algorithm/program which I called BMPGlyph and works on both Linux and Windows. I uploaded a beta version which you can download by clicking here. Just read the instructions in the zipfile.
I will also try to make an algorithm that extracts the heightmap from an autostereogram. It will be very helpful those people that cannot see autostereograms. :-)
Sunday, February 24, 2008
DizzyDiff v1.5.5
Thursday, February 21, 2008
Useful Firefox Add-Ons
It's really bad when a powerful company wants to put standards on everyone else. It usually has negative effects on everybody else, especially if those standards are worst that the standards that the others has agreed upon collectively. Why would someone then use Internet Explorer? A browser that while it doesn't follow standards, creates many security risks when used by an average user. Still not convinced? Try reading some of these pages.
On the other hand Firefox is faster, safer and extremely customizable.
Inside Firefox's Tools menu you can find the Add-Ons panel. Open it and the click where it says: "Get Extensions". It will send you to a webpage listing hundreds of Add-Ons. Try browsing the categories and I am sure that you will find many of them useful. Also, Opera users will find Add-Ons that add functions like Speed-Dial etc to Firefox.
Here is a list of the Add-Ons that I have installed. Don't forget that if you want to make full use of them you have to explore their preferences and customize them.
- Adblock Plus: A MUST-HAVE Add-On. Install it and after subscribing (completely free) to some lists and you can say goodbye to almost all advertisements! I have subscribed to:
- Cache View: Add some options to the right click menu that make easy viewing for cached version of any website by Google, Web Archive etc... This means that you can view older versions of a temporarily or permanently down website.
- CustomizeGoogle: Allows you to customize many aspects of Google and it's services. One feature I really liked is that it switches to a secure connection (https) when possible (https is supported by Gmail, Google Documents and other services too). Also view the introduction movie which is available at their website.
- del.icio.us Bookmarks: Makes bookmarking with del.icio.us very easy. I hope you know what delicious is... ;-)
- Download Statusbar: An extra statusbar that replaces the downloads window.
- external IP: Shows your current IP and alerts you when it changes.
- Fast Video Download: Allows you to easily download videos (in flv format) from Youtube and other sites.
- FasterFox: It's supposed to make firefox faster. I just use it because it adds a page load timer on the statusbar. Sometimes I like playing with its settings too. But I haven't noticed a change in Firefox's speed.
- Google ToolBar for Firefox: Search using Google easily. It also has many other functions as well.
- Greasemonkey: Add more functionality to some webpages by installing some user scripts.
- HackBar: "This toolbar will help you in testing SQL injections, XSS holes and site security."
- Informational Tab: Add a progressbar (which I'm still unable to see because of my dark theme) and a tiny preview to the tab buttons.
- ReloadEvery: Reloads webpages periodically. Very useful if you hate been logged out of forums after been inactive for some time.
- Smart Middle Click: "By default, middle clicking on javascript links in Firefox opens a blank tab instead of following the link. This extension fixes that problem and lets middle clicks open simple javascript links in new tabs."
- Snap Links: Allows you to multiple links at once by drawing a rectangle around them.
- Tab Scope: Allows you to preview open tabs in a mini window when by putting your mouse over the tab button.
- Table2Clipboard: Allows you to copy to the clipboard the selected columns/rows of a table.
- Tabs Open Relative: Very useful Add-On! It makes any new tab appear next to the current tab instead of the far right of the tab bar. It really helps if you usually work with many tabs of different sites at the same time (like me :-P).
- User Agent Switcher: Makes Firefox send fake User-Agent information in HTTP requests.
PS: If you know any other useful Add-On then you may leave a comment suggesting it!
Monday, February 18, 2008
Memory efficient Brainfuck tables
The table has the following structure:
0 I I D (0) (1) (2) (3) ...
^
For a table with n cells you will need n+4 cells on the Brainfuck array.
( n <= cell size) We start from where the ^ points. The first cell should be empty (zero). The second and third cells contain the index that we want to write to or read from. The fourth cell contains the data that we want to store in the table. If we want to read from the table then we should leave this cell empty and it will later carry the read data. The next cells (0) (1) ... (n-1) are the actual data stored in the table. We'll call the I I D part the "head". It works like this: Move the head to the right place: (the head moves to the right by moving the data of the next cell to the head's preceding empty cell, then moving each of the I I D cells one place to the right creating a new empty preceding cell.)
We will move the head to the right to the correct index:
>[
>>>[-<<<<+>>>>]
<
If we want to write something then we should carry the D cell too: [->+<]
<[->+<]
<[->+<]
>-]
Now we may read:
>>>[-<+<<+>>>]<<<[->>>+<<<]>
Or we may write:
>>>[-]<[->+<]<
Now we will return the head to it's initial position:
[
[-<+>]
If we have read something then we need to carry the D cell too: >[-<+>]<
<<<[->>>>+<<<<]
>>-]
<<
After a successful read the I I cells should be empty while the D cell will hold the read data.
After a successful write the I I D cells should be empty.
The pointer ends at the some place where it was before we used the algorithm, that is to the left of the first I cell.
Here is the code uncommented:
Write:
>[>>>[-<<<<+>>>>]<[->+<]<[->+<]<[->+<]>-]
>>>[-]<[->+<]<
[[-<+>]<<<[->>>>+<<<<]>>-]<<
Read:
>[>>>[-<<<<+>>>>]<<[->+<]<[->+<]>-]
>>>[-<+<<+>>>]<<<[->>>+<<<]>
[[-<+>]>[-<+>]<<<<[->>>>+<<<<]>>-]<<
Saturday, February 16, 2008
Tiny Lua Distribution
Monday, February 11, 2008
SFTM (mastermind solver)
[EDIT] Version 2.0 is out! Read the related post.
Sunday, February 10, 2008
Strange Xmoto physics
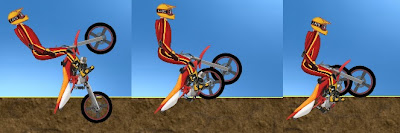
Friday, February 8, 2008
Sagem Fast 800 on Ubuntu
For instructions in Greek go here. If you encounter any problems during the installation insert the Ubuntu CD and then restart the installation. (tip by DimBiC)
Και στα Ελληνικά:
Επιτέλους το Sagem Fast 800 δουλεύει και στo Ubuntu! Ακολουθήστε τις οδηγίες που θα βρείτε εδώ. Αν αντιμετωπίσετε κάποιο πρόβλημα κατά την εγκατάσταση τότε τοποθετήστε το CD του Ubuntu και ξαναξεκινήστε την εγκατάσταση. (συμβουλή του DimBiC)
Wednesday, February 6, 2008
How to configure Compiz in Gutsy
The good thing is that Ubuntu Gutsy Gibbon comes with Compiz pre-installed. The bad thing is that they did not include the settings manager, leaving you with only three preset configurations (found in System->Preferences->Appearance->Visual Effects).
If you want more control over compiz (compiz is capable for much more things than those found in the "Extra" preset) will have to install compizconfig-settings-manager. To do this, use a console (Applications->Accessories->Terminal) to execute this command:
sudo apt-get install compizconfig-settings-manager
(or use System->Administration->Synaptic Package Manager to find and install the package)
Now go to System->Preferences->Appearance. It should contain one more option named "Custom":
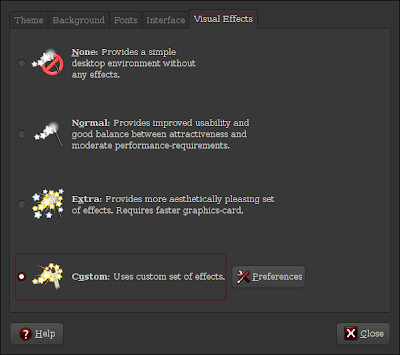
If you have no idea how to configure them you can download my settings by clicking here. I use mostly the fire effect (because it matches with Ubuntu SE). If you move the pointer to the upper left corner of the screen you will get thumbnails of all open windows in all desktops. There are 4 desktops which form a cube that you are inside it. By touching the left or the right screen edge you can move to the next or previous desktop. Moving the pointer to the upper right corner will show you the four desktops. Take a look at this post as it contains two videos demonstrating the above.
To import my settings follow these steps:
- System->Preferences->Appearance
- Click the Preferences button on the Visual Effects tab.
- In CompizConfig Settings Manager click on Preferences.
- Create a new profile by clicking on the + symbol.
- Click Import and select the downloaded file.
Sunday, February 3, 2008
Medibuntu
- Click on Applications->Accessories->Terminal.
- Now copy, paste and execute the following line:sudo wget http://www.medibuntu.org/sources.list.d/hardy.list -O /etc/apt/sources.list.d/medibuntu.list ; wget -q http://packages.medibuntu.org/medibuntu-key.gpg -O- | sudo apt-key add - && sudo apt-get update
- Run Synaptic (System->Administration->Synaptic Package Manager).
- Click Reload to get the packages from the newly installed repository.
- Click on Origin (it's one of the buttons at the bottom left part of the window).
- Select the first Medibuntu repository, then the second etc to view the packages they include. And install whatever you need.
- hot-babe: Read the description and don't forget to add it to the Startup programs (System->Preferences->Sessions). Just make sure that you use the following command to run it or the babe will suck on your CPU: hot-babe -i -n 10
(But even if you use this command the babe uses the CPU a lot and it's a bit buggy especially if used with Compiz, but I suggest that you try her ;-) ... )
Here is a photo of the babe (fully dressed) :-P - libdvdcss2: Allows you to play encrypted DVDs. Also search for and install libdvdread3 using Synaptic.
- Google Earth
- Skype
- non-free-codecs: To play some video files that no open source decoder exists for them.
Sunday, January 27, 2008
Ubuntu Satanic Edition and Compiz
Sunday, January 20, 2008
SEcure PUblic Key TRAnsfer
- Each of the two parts generate a pair of public - private keys.
- The two parts exchange their public keys over a possibly insecure communication channel.
- Now if one part wants to send a message to the other it simply encrypts it with the public key of the other part and then sends it. An eavesdropper cannot decrypt this message because he has not got the private key needed.
- Bob generates a keypair. (a public and a private key) and sends his public key to Alice.
- Mario, who is an employer in Bob's ISP, has already generated a keypair and while the packet with Bob's key is transient he replaces Bob's key with his own (he also notes down Bob's public key).
- Alice receives Mario's public key but she believes it's Bob's.
- Alice generates a keypair and sends her public key to Bob but Mario replaces it with his own, just like he did with Bob's.
- Now Bob wants to send some data to Alice. He encrypts it with Mario's key believing that it's Alice's key and sends the encrypted data.
- The encrypted data is peaked by Mario who is able to decrypt them with his own private key. He then re-encodes them with Alice's correct public key and passes them on Alice.
- The same thing happens when Alice replies to Bob. Mario is able to eavesdrop on the connection even though both part's think that is secure.
I tried to understand the algorithm but I couldn't! And I think that it's too complex for no reason... They could use this protocol to achieve the same thing:While data messages are being exchanged, either Alice or Bob may run SMP to detect impersonation or man-in-the-middle attacks. As above, all exponentiations are done modulo a particular 1536-bit prime, and g1 is a generator of that group. All sent values include zero-knowledge proofs that they were generated according to this protocol, as indicated in the detailed description below.
Suppose Alice and Bob have secret information x and y respectively, and they wish to know whether x = y. The Socialist Millionaires' Protocol allows them to compare x and y without revealing any other information than the value of (x == y). For OTR, the secrets contain information about both parties' long-term authentication public keys, as well as information entered by the users themselves. If x = y, this means that Alice and Bob entered the same secret information, and so must be the same entities who established that secret to begin with.
- Bob knows X and Alice knows Y and they want to compare them.
- Bob sends some random data to Alice. Alice does the same with some other random data.
- Bob appends X to the end of the data he received and then computes the hash of the new data. Alice appends Y to the end of the data she sent to Bob and computes the hash of the new data. Bob sends the hash to Alice. Alice checks whether the two hashes are the same.
- Alice appends Y to the end of the data she received and then computes the hash of the new data. Bob appends X to the end of the data he sent to Alice and computes the hash of the new data. Alice sends the hash to Bob. Bob checks whether the hashes are the same.
- H(x) is the hash of x. Any hash function can be used. Take a look at MD5.
- E(x,k) is the symmetrically encrypted data of x with k used as the encryption key.
- DE(x,k) is the symmetrically decrypted data of x with k used as the decryption key.
- PK is the public key of the sender.
- RDP means Random Data Padding.
- & is the concatenation symbol (VB-style :-P).
- len(x) is the length in bytes of x.
- D: The Data packet. It is composed of 4 parts: len(RDP) & RDP & H(K) & E(PK & data,k)
- A: The Acknowledge packet. It is composed of 3 parts: RDP & H(D)
- K: The Key packet. It is composed of 4 parts: RDP & H(A) & k
- Exchange public keys with the other part and start an encrypted connection.
- If you are the part that initiated the connection then set time1=0 time2=inf and jump to step 11.
- Generate a random key (k) for a symmetric encryption.
- Read data (data) from user.
- Calculate E(data,k) and H(K) (where K is the last K packet received).
- Note down the current time (time1).
- Send a D packet (with the structure described above).
- Wait for an A packet.
- Note down the current time (time2).
- Send a K packet containing the H(A) and the key (k) you used in step 5.
- Wait for a D packet and store the encrypted data (data) it contains.
- Note down the current time (time3).
- If (time2-time1)*2<=(time3-time2) then either there is a MITM or you connection is really unstable.
- Send an A packet containing H(K).
- Wait for a K packet and store the key (k) it contains.
- Show DE(data,k) to the user.
- Jump to step 3.
Popular Posts
-
This article probably also applies to: VGN-FZ38M Yeap I bought it. I though that since it had an NVidia card and it was Centrino based etc i...
-
It seems that the most popular article on this blog is " How to make portable applications " written back in 2007. Reading it aga...
-
The other day, while browsing Wikipedia, I wondered how hard it must be to have weird fetishes without being able to share them with someone...